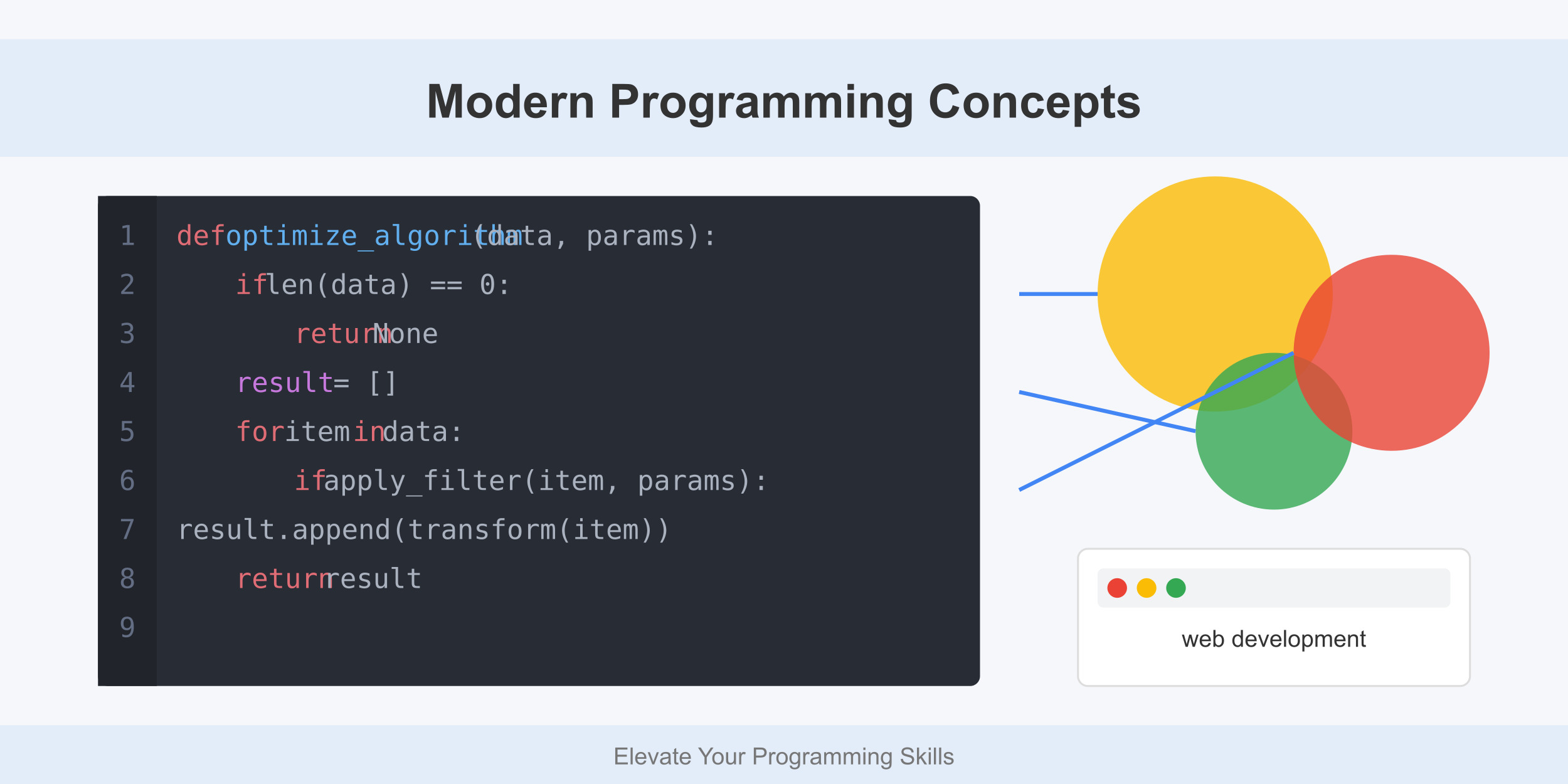
So, you’re gearing up for a technical interview, huh? Well, buckle up, ’cause it’s gonna be a ride. Whether you’re eyeing a sweet gig at one of those big tech companies or just wanna level up your programming game, you gotta know your data structures and algorithms. No two ways about it. These bad boys form the backbone of problem-solving in the coding world. Without ‘em, you’re basically trying to build a skyscraper on sand. Not a good look.
Why Data Structures and Algorithms Matter
Okay, so here’s the deal. Data structures are like the storage containers of the programming world. Think of ‘em like different kinds of boxes—some are better for quick lookups, some are great for sorting, others for fast inserts and deletions. Algorithms, on the other hand, are like the recipes. They tell you exactly how to mix your ingredients (data) to get the dish (solution) you want. And just like in cooking, the efficiency of your recipe matters—a lot.
Technical interviews? They’re all about testing how well you know your boxes and recipes. Let’s dive into some of the essential ones that pop up all the time in interviews.
Must-Know Data Structures
1. Arrays and Lists – The OGs
These are like the bread and butter of programming. You use ‘em for, well, everything. An array is a fixed-size collection of elements, while a list is dynamic and can change in size. Interviewers love asking questions that test your ability to manipulate these guys efficiently. Think things like reversing an array, rotating it, or merging two sorted lists.
2. Hash Tables – Speed Demons
Imagine you have a huge phonebook, and instead of flipping through pages, you can jump straight to a name in one go. That’s a hash table. They use a key-value pairing and some hashing magic to make lookups crazy fast. Interviewers love to throw problems at you that can be cracked with hash tables—like detecting duplicates, finding anagrams, or implementing a cache.
3. Stacks and Queues – The Organized Chaos
Stacks work like a stack of plates—you can only take the top one off. LIFO (Last In, First Out). Queues, on the other hand, work like a line at a coffee shop—first in, first out (FIFO). These data structures get tested in problems involving recursion, parsing expressions, or managing tasks.
4. Trees – Hierarchical Goodness
A tree is basically an upside-down family tree. Each node has children, and you traverse through it in different ways (inorder, preorder, postorder). Binary Search Trees (BSTs) are particularly hot in interviews—knowing how to insert, delete, and balance ‘em is a must.
5. Graphs – Networks Everywhere
Graphs represent relationships, like social networks, road maps, and even AI neural networks. There’s a lot of depth here, but breadth-first search (BFS) and depth-first search (DFS) are absolute must-knows for interviews. You’ll use ‘em to solve problems like shortest path, cycle detection, and connected components.
Must-Know Algorithms
1. Sorting Algorithms – Because Order Matters
Sorting is like cleaning up your messy room—it makes everything easier to find. Interviewers love classic sorting algorithms like:
- Bubble Sort (the slowpoke but easy-to-understand one)
- Merge Sort (efficient, uses divide and conquer)
- Quick Sort (fast and practical, used in real-world scenarios)
- Heap Sort (solid choice when dealing with priority queues)
2. Searching Algorithms – Finding a Needle in a Haystack
- Binary Search: Super fast if the data is sorted. Think of searching for a name in a phonebook by flipping straight to the middle instead of starting at page one.
- Linear Search: Simple, but slow. You just go through each item until you find what you need.
3. Dynamic Programming – The Brain-Buster
This is where things start getting tricky. DP problems are all about breaking things into smaller subproblems and storing results to avoid redundant work. Classic examples include:
- Fibonacci sequence (with memoization)
- Knapsack problem
- Longest Common Subsequence
If you wanna impress in an interview, mastering DP will give you serious street cred.
4. Greedy Algorithms – Grab What You Can
These algorithms make the best choice at every step without worrying about the future. Sometimes it works great (like Huffman coding), sometimes it backfires.
5. Graph Algorithms – Traversing the Web
- Dijkstra’s Algorithm: Used for shortest paths, like Google Maps figuring out the best route.
- A Algorithm*: Smarter pathfinding used in AI and gaming.
Pro Tips for Technical Interviews
- Practice Like a Maniac – Sites like LeetCode, HackerRank, and CodeSignal are your best friends. You won’t master this stuff overnight, but consistent practice makes a difference.
- Time & Space Complexity is Key – Knowing Big O notation will save your skin when an interviewer asks, “What’s the runtime of your solution?”
- Think Out Loud – Don’t just sit there and scribble. Interviewers wanna hear how you think, so talk through your approach.
- Test Edge Cases – Your solution might work for basic inputs, but what about empty arrays, huge numbers, or duplicate values? Always check!
- Stay Cool Under Pressure – Yeah, easier said than done. But seriously, even if you get stuck, stay calm and explain what you’re thinking.
Wrapping It Up
Look, mastering data structures and algorithms isn’t just about cracking interviews—it’s about becoming a better problem solver. Once you get the hang of ‘em, you’ll start seeing how they apply to real-world programming. Whether it’s optimizing an app’s performance or handling large datasets efficiently, this stuff is pure gold. Plus, who doesn’t wanna flex their skills and snag that dream job?
And hey, if you’re feeling stuck, don’t stress too much. Getting help—whether it’s through online resources, coding bootcamps, or even programming homework writing services—can be a game-changer. Keep grinding, and you’ll get there.
Author Bio:
This article was written by Warren a programming enthusiast and tech mentor working with New Assignment Help, dedicated to helping students and professionals ace their coding interviews. When not buried in code, they’re probably sipping on coffee and ranting about inefficient algorithms.