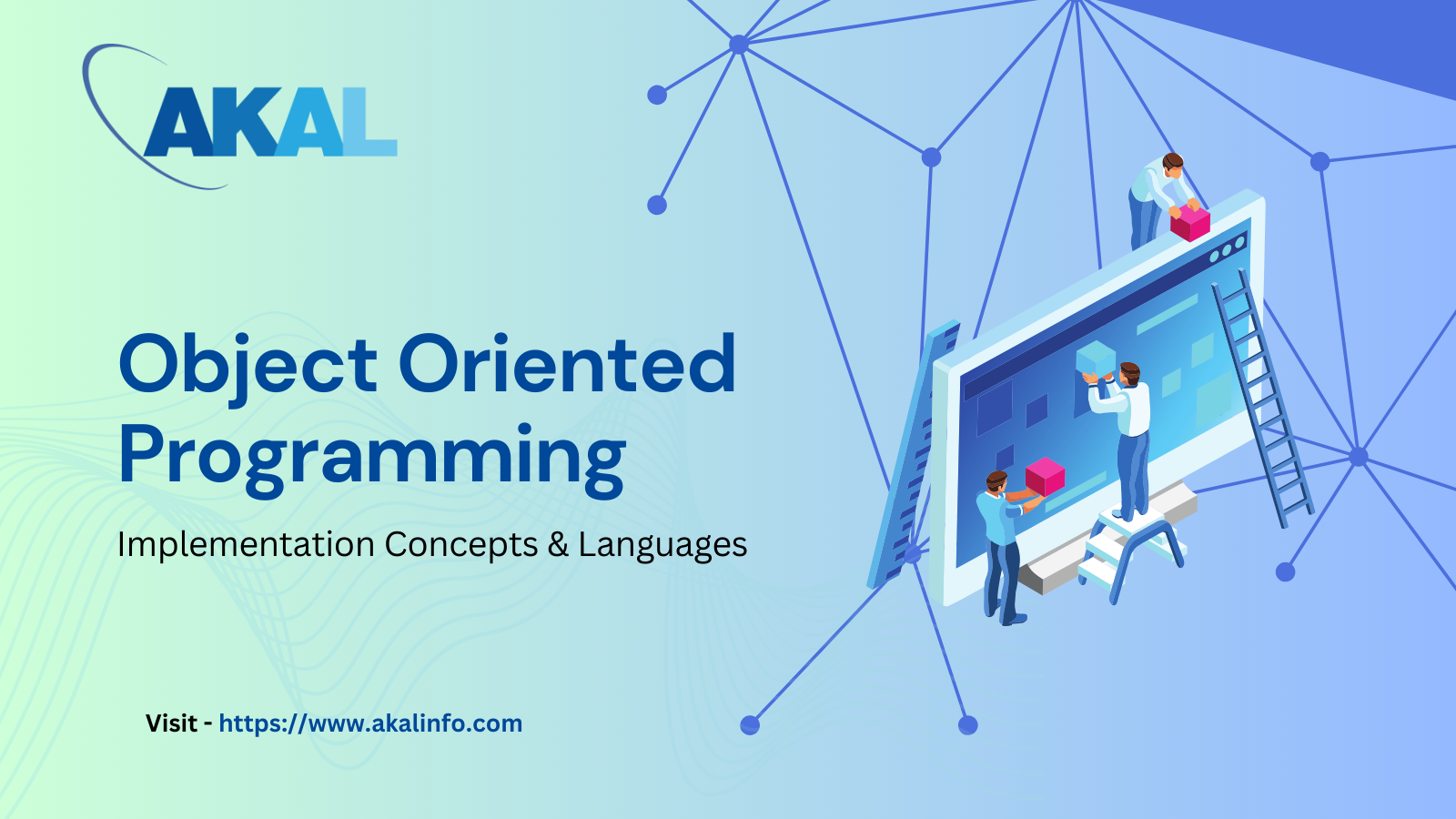
Object-Oriented Programming for Novices: A Beginner’s Primer
Object-oriented programming is the foundation of today’s software development. It’s a structured programming approach that is centered on objects-self-contained entities of data and functions. In order to understand how to construct scalable and maintainable software systems, any developer needs to grasp OOP.
What is Object-Oriented Programming?
OOP is a paradigm of programming wherein objects, containing data and behavior, are the basic building blocks. With each object being an instance of its class-a blueprint that defines an object’s name, data types, methods – is the basis of Classes and Objects. Java, Python, and Object-Oriented Programming C++ all implement principles of OOP to varying degrees.
Advantages of OOP:
- Code Reusability- Classes and objects can be reused in multiple projects
- Scalability- Can grow without changing already written code
- Maintainability- Updating and debugging becomes easy.
- Modularity- Big applications can be broken into small components.
The Four Pillars of OOP Explained
To become a master in OOP, first, learn the four main pillars:
Encapsulation:
Hides an object’s state internally and allows controlled access using methods.
For example, In C++, data members can be declared private, thus only accessible via public methods.
Inheritance:
Let a class acquire properties and behavior from another class.
Example: A Truck class which is extending a Vehicle class.
Abstraction
Identifies the basic attributes and excludes the information about how it would be done
Example: In Java, in abstract classes methods are declared, which a subclass has to override
Polymorphism
The same name method performs operation because of a condition at run-time due to calling object.
Example: Using the concept of overloading, computeArea () method of class Shape
OOP vs. Functional Programming: Comparative Study
Though these two paradigms are broadly used in the programming world, they are highly applied to completely different usage of programming:
Object Oriented Programming in Real Life
OOP is highly taken up in the market because it is flexible. The adoption of OOP is pretty diversified:
- Web Development: frameworks such as Django and Spring are implemented by using OOP.
- Game Development: Unity employs OOP in game development through modular code.
- Enterprise Software: large applications are coded with OOP for effective management.
- Mobile Applications: Native Android (Kotlin) and iOS (Swift) is implemented based on design principles of OOP
Most common OOP Design Patterns Any Programmer Must Know
Design patterns are tried, tested and most effective methods adopted for resolving often encountered problems, which commonly occur during the design of any software. Core OOP patterns:
- Singleton: It ensures that class has only single instance and so provides global point of access.
- Factory Method: Objects are instantiated without having to declare concrete classes.
- Observer: Introduces dependency, where one object notifies others about changes.
- Decorator: Introduces some new functionality on an object without modifying its design.
Advanced Object-Oriented Programming Concepts
Advanced topics for those experienced developers wanting to take knowledge to the next level:
Dynamic Binding: calls methods at runtime, not compile time.
Multiple Inheritance: Supported in C++ but causes many complexities including diamond problem.
SOLID Principles: Best practices to write clean and maintainable code.
Composition Over Inheritance: It advocates objects in objects rather than the very deep hierarchies of inheritance.
History and Evolution of Object-Oriented Programming
OOP has evolved a lot from the day it was invented:
- Early Days: Classes and objects were first invented in Simula 67.
- Emergence of Smalltalk: It spread around the world and brought OOP with a fully object-oriented environment.
- Acceptance in Mainstream: With languages like C++ and Java, OOP reached the masses.
- Contemporary Era: Other paradigms, functional programming for example, are married to OOP to give hybrid approaches.
Writing Clean, Maintainable OOP Code
- Maintain clean, maintainable OOP code: A long-term success for a project.
- Implementation of Naming Conventions: Use clear class, method, and variable names.
- Encapsulation: Private access modifiers can be applied to make the data of objects private
- Limit Class Responsibilities: SRP
- Refactor Regularly: Clear mess and eliminate duplication.
- Write Tests: Each module should be reliable with unit tests.
Object-Oriented Programming in Contemporary Software Development
Modularity and scalability make OOP a need in contemporary software development:
- Microservices Architecture: Objects are the modular units of services.
- Cloud Computing: OOP provides the features of reuse and flexibility to code; one can therefore create cloud-based applications.
- AI and Machine Learning: Using an OOP-based framework such as TensorFlow in conjunction with pipeline for data
- Object Oriented Programming in Other Languages: A Comparison Still, each does so in their way but having an advantage on their part
Conclusion
Object-Oriented Programming is the foundation of software development today. So long as a developer has all the principles and applications of it, he could come up with robust, maintainable, and scalable systems. Be it Object-Oriented Programming in C++, Java, or even Python, the paradigm’s versatility ensures relevance in the constantly moving landscape of tech.