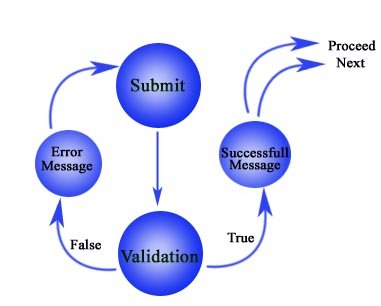
Introduction
Ensuring secure and error-free form submissions is crucial for any website that collects user data. One of the most important aspects of form security is email validation. Improper email validation can lead to spam, incorrect data storage, and even security vulnerabilities. In this guide, we will explore PHP Email Validation: Best Practices to Verify and Sanitize User Emails to help developers implement effective email verification strategies.
Why Email Validation is Important
Email validation serves multiple purposes, including:
- Preventing invalid email submissions: Ensures users enter properly formatted emails.
- Reducing spam: Filters out bots and spam email addresses.
- Enhancing security: Protects against SQL injection and other malicious attacks.
- Improving user experience: Helps users enter correct email addresses without frustration.
Different Methods for PHP Email Validation
There are multiple ways to validate email addresses in PHP, including built-in functions, regular expressions, and third-party libraries.
1. Using filter_var() Function
PHP provides a built-in function called filter_var()
to validate emails efficiently.
$email = "[email protected]";
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo "Valid email address.";
} else {
echo "Invalid email address.";
}
Pros:
- Simple and built-in
- Reliable for basic validation
- No external dependencies
Cons:
- Does not check if the email exists or is active
2. Using Regular Expressions (Regex)
A more customized validation approach is to use regular expressions (regex) to match email patterns.
$email = "[email protected]";
$pattern = "/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/";
if (preg_match($pattern, $email)) {
echo "Valid email address.";
} else {
echo "Invalid email address.";
}
Pros:
- More control over validation rules
- Can block specific unwanted email formats
Cons:
- Can be complex to write and maintain
- Still does not verify email existence
3. Verifying Email Domain via DNS Records
Checking an email’s domain against DNS records helps verify its legitimacy.
$email = "[email protected]";
list($user, $domain) = explode('@', $email);
if (checkdnsrr($domain, 'MX')) {
echo "Domain is valid.";
} else {
echo "Invalid domain.";
}
Pros:
- Helps confirm if an email domain exists
Cons:
- Does not confirm if the email address itself is active
- Some free email providers might block DNS lookups
4. Using Third-Party API for Advanced Validation
For comprehensive validation, third-party services like ZeroBounce, Hunter.io, or Mailgun API offer email verification solutions.
Example using Mailgun API:
$apiKey = "your_api_key_here";
$email = "[email protected]";
$ch = curl_init("https://api.mailgun.net/v4/address/validate?address=$email");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, "api:$apiKey");
$response = curl_exec($ch);
curl_close($ch);
$data = json_decode($response, true);
if ($data['is_valid']) {
echo "Valid email.";
} else {
echo "Invalid email.";
}
Pros:
- Provides real-time validation
- Can check if an email exists
- Reduces spam and fake emails
Cons:
- Requires API integration
- May have additional costs
Sanitizing Email Input for Security
Besides validation, it is important to sanitize user input to prevent security risks.
$email = filter_var($_POST['email'], FILTER_SANITIZE_EMAIL);
This removes unnecessary characters and prevents malicious code injection.
Implementing Email Validation in a Contact Form
Here’s how to integrate PHP email validation into a simple contact form:
HTML Form:
<form method="POST" action="process.php">
<input type="email" name="email" required>
<button type="submit">Submit</button>
</form>
PHP Processing Script (process.php):
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$email = filter_var($_POST['email'], FILTER_SANITIZE_EMAIL);
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
if (checkdnsrr(explode('@', $email)[1], 'MX')) {
echo "Email is valid and domain exists.";
} else {
echo "Invalid domain.";
}
} else {
echo "Invalid email format.";
}
}
Conclusion
Proper PHP email validation is essential for secure and error-free forms. Using built-in functions like filter_var()
, regex, DNS lookups, and third-party APIs can enhance the validation process. Additionally, sanitizing inputs prevents security vulnerabilities. By implementing these methods, developers can ensure reliable email verification and improve user experience on their websites.