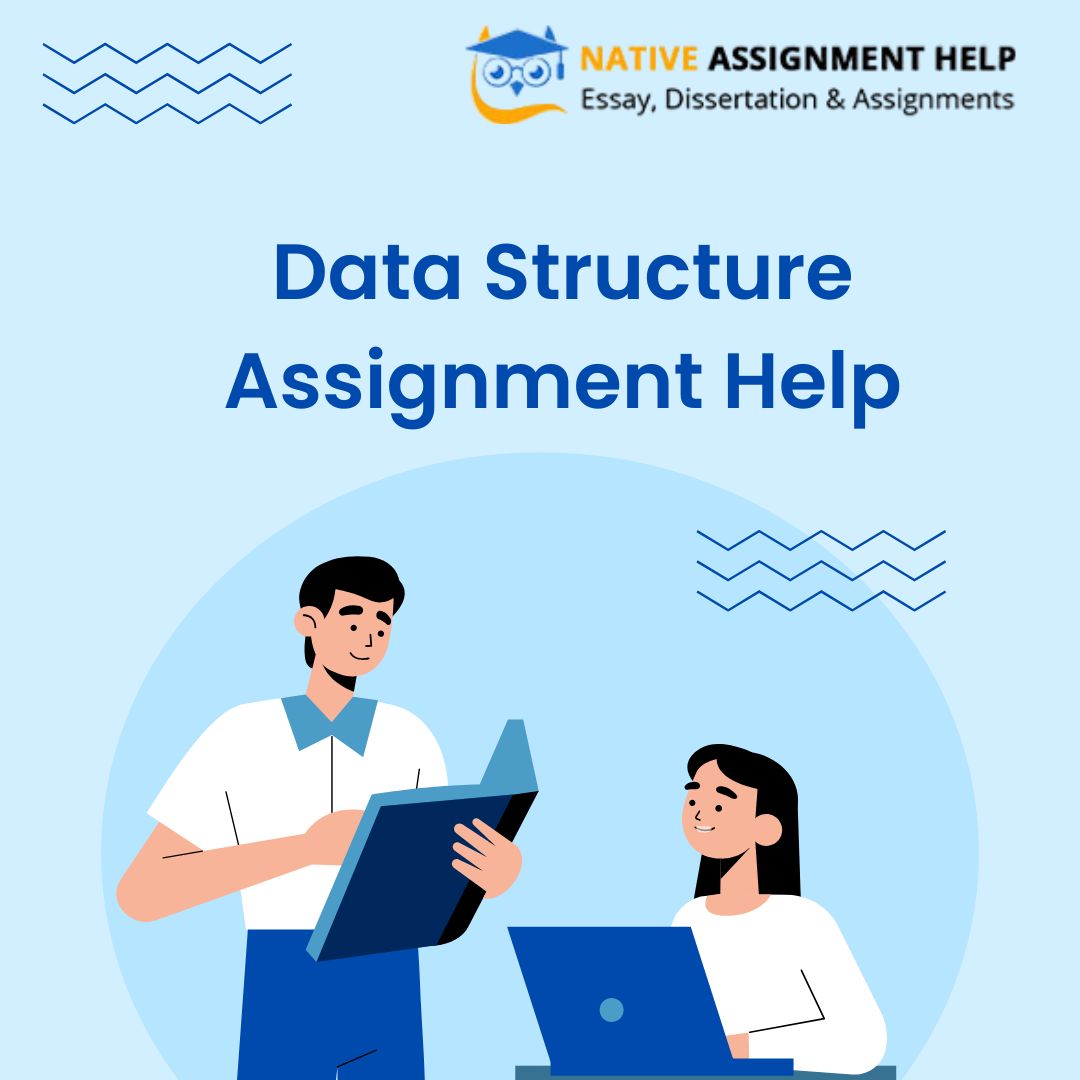
Introduction
Whether you’re a computer science student tackling assignments or a budding software developer optimizing algorithms, one concept you’ll consistently encounter is time complexity analysis. It’s the bedrock of efficient programming—ensuring that your solutions not only work but scale with larger inputs.
But what does time complexity really mean? And how can you apply it to your data structure solutions? If you’re seeking data structure assignment help, mastering time complexity is essential to crafting high-performance code. This article breaks down the fundamentals of time complexity, offers methods to analyze it, and teaches you how to use it to your advantage when designing or evaluating data structures.
What Is Time Complexity?
Time complexity refers to the computational complexity that describes the amount of time an algorithm takes to run as a function of the size of its input. It gives you a high-level understanding of how your code performs, especially when dealing with large data sets.
Rather than measuring time in seconds or milliseconds, time complexity uses Big O notation to express the growth rate of an algorithm’s running time.
For instance:
- O(1) means constant time—no matter how large the input, execution time stays the same.
- O(n) means linear time—performance grows proportionally with input size.
- O(n²) means quadratic time—execution time squares as input grows.
Why Is Time Complexity Important?
In assignments and real-world applications alike, it’s not enough for your code to produce the correct result—it must also do so efficiently.
Here’s why time complexity analysis matters:
- Scalability: An algorithm that works fine for 10 items may become unusable for 10,000 if it’s inefficient.
- Performance optimization: Choosing the right data structure often depends on its time complexity for various operations (e.g., search, insert, delete).
- Competitive coding and exams: Efficient algorithms earn better scores and can handle edge cases under tight time constraints.
- Code quality: Time complexity analysis reflects how deeply you understand your solution’s efficiency.
Common Time Complexities in Data Structures
Let’s review typical operations and their time complexities across popular data structures.
Operation | Array | Linked List | Stack/Queue | Hash Table | Binary Search Tree |
---|---|---|---|---|---|
Access | O(1) | O(n) | O(n) | O(1) | O(log n) (avg case) |
Search | O(n) | O(n) | O(n) | O(1) (avg) | O(log n) (avg case) |
Insertion | O(n) | O(1) | O(1) | O(1) | O(log n) |
Deletion | O(n) | O(1) | O(1) | O(1) | O(log n) |
These complexities assume average cases. Worst-case scenarios (e.g., an unbalanced binary tree) can degrade performance drastically—emphasizing the need to understand both theory and implementation.
How to Evaluate Time Complexity
1. Identify Input Size (n)
Start by determining what represents the input size in your problem. Is it:
- The number of elements in an array?
- The number of nodes in a graph?
- The depth of a tree?
The symbol “n” typically represents input size. If multiple inputs exist, you might use multiple variables like “m” and “n.”
2. Count Basic Operations
Break your algorithm down into basic steps—assignments, comparisons, loops, and function calls. Focus on how many times each operation runs relative to input size.
Example:
def print_pairs(arr): for i in range(len(arr)): for j in range(len(arr)): print(arr[i], arr[j])
This double loop results in O(n²) time complexity because for each of the n
elements, it loops over n
elements again.
3. Analyze Loops and Recursions
Loops are the most straightforward source of time complexity:
- A single loop running
n
times = O(n) - A nested loop where each inner loop runs
n
times = O(n²) - A loop halving the input size each time (like binary search) = O(log n)
Recursive functions require closer examination. A recursive algorithm like merge sort:
def merge_sort(arr): if len(arr) > 1: mid = len(arr) // 2 L = arr[:mid] R = arr[mid:] merge_sort(L) merge_sort(R) merge(L, R)
This follows the recurrence: T(n) = 2T(n/2) + O(n), which solves to O(n log n).
Real-World Examples of Time Complexity in Data Structures
1. Searching in Arrays vs. Hash Tables
Suppose you’re frequently searching for items. A linear search in an array gives you O(n) time, while a hash table lookup provides O(1) on average.
Key takeaway: If your assignment help involves frequent lookups, a hash table is your best friend.
2. Stack Operations in O(1)
Stacks are great for problems like expression evaluation or reversing strings. All core operations (push
, pop
, peek
) are O(1), making them highly efficient for such use cases.
3. Binary Trees and Logarithmic Efficiency
Balanced binary search trees (like AVL or Red-Black trees) allow O(log n) insertion and search—ideal for maintaining sorted data dynamically.
However, an unbalanced tree may degrade to O(n) in worst cases, such as inserting sorted data into a basic BST.
Best Practices for Evaluating Time Complexity
1. Use Big O for Worst Case Analysis
Even if average performance is acceptable, always plan for the worst-case scenario. Algorithms must handle peak stress without crashing or slowing to a crawl.
2. Consider Hidden Constants in Real Applications
Although Big O ignores constants (e.g., O(2n) becomes O(n)), in practical settings, these constants matter. An O(n) algorithm with a large constant may perform worse than a better-implemented O(n log n) algorithm for small datasets.
3. Choose the Right Data Structure
Time complexity should guide your choice. Need fast insertions and deletions? Use a linked list. Want fast lookups? Use a hash table. Maintaining order? A tree might be best.
4. Optimize Hot Paths
Focus on optimizing parts of the code that run most frequently or affect performance most—these are called hot paths. Reducing complexity here can drastically improve overall performance.
Visualizing Time Complexity
Here’s a general graph of how time complexity grows:
- O(1) – constant (best performance)
- O(log n) – logarithmic
- O(n) – linear
- O(n log n) – linearithmic
- O(n²) – quadratic (gets slow quickly)
- O(2^n) – exponential (unusable for large n)
- O(n!) – factorial (combinatorial explosion)
In assignment grading or production-level systems, your goal should be to stay in the O(1) to O(n log n) range whenever possible.
Conclusion
Understanding and applying time complexity analysis is a non-negotiable skill for anyone working with data structures. It goes beyond academic theory—helping you build faster, smarter, and more scalable solutions. Whether you’re debugging a slow loop, deciding between an array and a hash map, or preparing for a coding interview, knowing the cost of every operation puts you in control.
The next time you write an algorithm, don’t just ask if it works—ask how well it works as n grows. That single shift in perspective can elevate your entire approach to data structure design and evaluation.